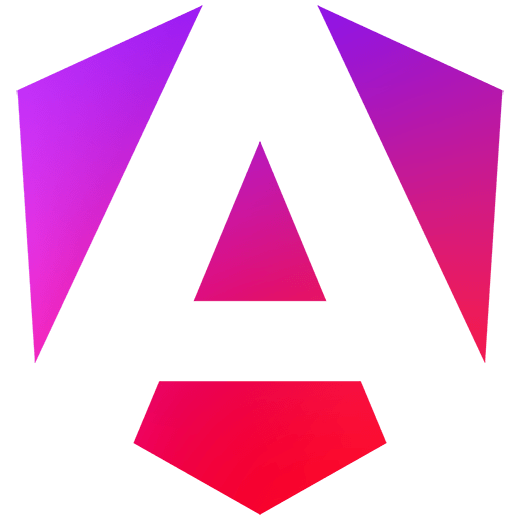
Angular中文博客
分享让你更聪明
在 Angular 的 SSR(Server-Side Rendering)开发中,如果你希望某段代码只在浏览器环境中运行,可以通过检测当前的运行环境来实现。Angular 提供了 isPlatformBrowser
和 isPlatformServer
这两个工具函数来帮助你判断当前代码是在浏览器端还是服务器端执行。
以下是一个示例,展示如何在 Angular 中确保某段代码只在浏览器环境中运行:
导入必要的工具函数:
首先,你需要从 @angular/common
中导入 isPlatformBrowser
和 PLATFORM_ID
,并从 @angular/core
中导入 Inject
和 PLATFORM_ID
。
import { isPlatformBrowser } from '@angular/common';
import { Inject, Injectable, PLATFORM_ID } from '@angular/core';
在组件或服务中使用:
你可以在组件或服务中使用 isPlatformBrowser
来检测当前环境,并决定是否执行某段代码。
@Injectable({
providedIn: 'root'
})
export class SomeService {
constructor(@Inject(PLATFORM_ID) private platformId: Object) {}
someMethod() {
if (isPlatformBrowser(this.platformId)) {
// 这段代码只会在浏览器环境中执行
console.log('这段代码只在浏览器中运行');
// 例如,操作 DOM 或使用浏览器特有的 API
}
}
}
或者在组件中使用:
import { Component, Inject, PLATFORM_ID, OnInit } from '@angular/core';
import { isPlatformBrowser } from '@angular/common';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
constructor(@Inject(PLATFORM_ID) private platformId: Object) {}
ngOnInit() {
if (isPlatformBrowser(this.platformId)) {
// 这段代码只会在浏览器环境中执行
console.log('这段代码只在浏览器中运行');
// 例如,操作 DOM 或使用浏览器特有的 API
}
}
}
避免在服务器端运行浏览器特有的代码:
通过这种方式,你可以确保某些代码(如操作 DOM 或使用 window
、document
等浏览器特有的对象)不会在服务器端执行,从而避免 SSR 中的错误。
注意事项
isPlatformBrowser
是一个轻量级的检查,但在频繁调用的场景中,仍需要注意性能影响。通过这种方式,你可以有效地控制代码在 SSR 环境中的执行逻辑,确保浏览器特有的代码只在客户端运行。